Best Practices for Web Development
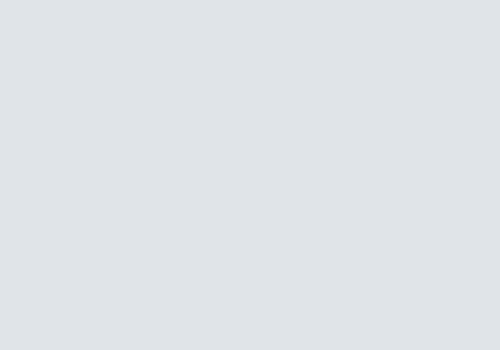
When it comes to web development, adhering to best practices is essential for creating high-quality, reliable, and scalable websites. Whether you’re a beginner or an experienced developer, following these best practices can help you improve your workflow and ensure that your web projects stand the test of time.
1. Write Clean, Maintainable, and Semantic Code
Why It Matters:
Clean, maintainable code is the backbone of any successful web project. It makes debugging easier, improves collaboration with other developers, and ensures the website can be updated or modified without introducing issues down the road.
Best Practices:
-
Use Descriptive Naming Conventions: Always name variables, functions, and files in a way that clearly describes their purpose. For example, instead of using
x
for a variable, use something descriptive liketotalPrice
oruserData
. -
Comment Your Code: Add comments to explain the purpose of complex code snippets. Avoid over-commenting simple code, but make sure anything complex is well-documented.
-
Follow a Consistent Coding Style: Adhere to a consistent coding style, such as proper indentation, spacing, and casing. Tools like Prettier or ESLint can help enforce these styles automatically.
-
Avoid Code Duplication: Reuse code and components where possible, especially when using modern JavaScript frameworks like React or Vue.js. This can be done through functions, components, and modules.
2. Prioritize Mobile-First Design
Why It Matters:
In 2025, mobile devices account for a significant portion of internet traffic. A mobile-first approach ensures that your website will be optimized for smaller screens first, then scaled up for larger devices. This approach enhances user experience (UX) and makes your site more accessible across devices.
Best Practices:
-
Responsive Design: Use CSS media queries to adjust the layout based on screen size. For example, use flexible grids, images, and fluid layouts that adapt seamlessly across different devices.
-
Mobile-First CSS Frameworks: Consider using frameworks like Bootstrap or Foundation, which are designed with a mobile-first approach and provide pre-built, responsive grid systems and components.
-
Simplify Navigation: Mobile users often rely on touch interfaces, so make navigation menus larger, simpler, and easier to interact with.
-
Prioritize Performance: Mobile devices generally have less processing power, so ensure your website loads quickly and doesn’t overwhelm the user with large assets or complex animations.
3. Optimize Website Performance
Why It Matters:
Website performance is a critical factor in user experience. Slow-loading websites can lead to high bounce rates and poor search engine rankings. Optimizing performance is a best practice that can have a massive impact on the usability and success of your website.
Best Practices:
-
Minimize HTTP Requests: Reduce the number of elements on a page (like images, scripts, and CSS files) to decrease the number of requests. Tools like Webpack or Parcel can help bundle files together.
-
Optimize Images: Compress images without compromising quality using tools like ImageOptim or TinyPNG. You can also use modern formats like WebP to reduce file sizes.
-
Use Lazy Loading: Load images and videos only when they’re in the viewport (visible part of the screen). This technique helps speed up initial page load times.
-
Minify CSS, JavaScript, and HTML: Minification removes unnecessary characters (like whitespace and comments) from your code, reducing file sizes and speeding up load times.
-
Enable Caching: Use caching to store static resources locally on the user’s device, so they don’t have to be downloaded every time they visit your site.
4. Ensure Cross-Browser Compatibility
Why It Matters:
Your website needs to work consistently across a variety of browsers and devices. This is crucial to reach a wider audience and ensure that no user is left with a broken experience.
Best Practices:
-
Test on Popular Browsers: Ensure your website works seamlessly on Chrome, Firefox, Safari, Edge, and other major browsers. Tools like BrowserStack and CrossBrowserTesting can help you test on different platforms.
-
Use CSS Vendor Prefixes: Some CSS properties may not be fully supported across all browsers. Use vendor prefixes (e.g.,
-webkit-
for Safari) or rely on Autoprefixer, a tool that adds necessary prefixes automatically. -
Graceful Degradation vs. Progressive Enhancement: Design for the latest browsers first (progressive enhancement) but ensure the site degrades gracefully on older browsers (graceful degradation).
5. Focus on Security
Why It Matters:
Security should be a top priority for any web developer. Data breaches, hacking, and security flaws can damage your reputation and cause users to lose trust in your site.
Best Practices:
-
Use HTTPS: Always secure your website with SSL/TLS encryption (HTTPS). This not only ensures that user data is transmitted securely but also improves SEO rankings.
-
Validate User Inputs: Always sanitize and validate user inputs to prevent malicious data (e.g., SQL injection, XSS attacks). Use built-in validation libraries like Joi for Node.js or Django Forms for Python.
-
Use Content Security Policy (CSP): A CSP can prevent cross-site scripting (XSS) attacks by specifying which sources are allowed to load content on your site.
-
Regularly Update Dependencies: Keep all libraries and frameworks up to date with the latest patches. This will protect your site from known vulnerabilities. Use tools like npm audit to check for outdated or insecure dependencies.
6. Use Version Control (Git)
Why It Matters:
Version control is essential for tracking changes, collaborating with other developers, and rolling back to previous versions if needed. Git is the most widely used version control system, and GitHub, GitLab, and Bitbucket are some of the most popular hosting platforms.
Best Practices:
-
Commit Frequently: Make commits regularly with descriptive messages. This ensures you can track changes over time and roll back to a specific version if necessary.
-
Branching Strategy: Use branches for new features, bug fixes, or experiments. Keep your
main
branch clean and stable, and merge changes back when they’re ready. -
Use Pull Requests: If you’re working in a team, use pull requests to review and discuss code before merging. This improves code quality and allows for peer feedback.
7. Prioritize Accessibility (a11y)
Why It Matters:
Web accessibility ensures that everyone, including people with disabilities, can use your website. In 2025, accessibility is not only a legal requirement in many countries, but it’s also crucial for creating an inclusive user experience.
Best Practices:
-
Use Semantic HTML: Properly use HTML tags like
<header>
,<footer>
,<article>
, and<nav>
to help screen readers interpret your site’s structure. -
Keyboard Navigation: Ensure that all interactive elements (like buttons, forms, and links) are fully accessible using only the keyboard.
-
Color Contrast: Make sure there’s enough contrast between text and background colors for users with visual impairments. Tools like WebAIM’s Contrast Checker can help.
-
Add Alt Text to Images: Provide descriptive alt text for all images, so users with screen readers can understand the content.
8. Keep User Experience (UX) in Mind
Why It Matters:
A great user experience is essential for user retention and satisfaction. A well-designed, easy-to-use website keeps users engaged and encourages them to return.
Best Practices:
-
Intuitive Navigation: Keep the navigation simple and easy to use. Use clear labels, logical hierarchies, and a search function.
-
Fast Load Time: A slow website can frustrate users. Optimize your website’s performance to keep loading times to a minimum.
-
Mobile Usability: Ensure that your website is just as functional and easy to use on mobile devices as it is on desktop computers.
-
Test with Real Users: Regularly test your website with real users to identify pain points, broken links, and confusing elements that could hinder the experience.
9. Optimize SEO (Search Engine Optimization)
Why It Matters:
SEO helps your website rank better in search engine results, making it easier for users to find your site. Good SEO practices not only improve visibility but also contribute to a better user experience.
Best Practices:
-
Use Proper HTML Tags: Make sure to use proper heading tags (
<h1>
,<h2>
, etc.) to define your page structure. This helps search engines understand your content. -
Optimize Meta Tags: Write clear, concise meta descriptions and title tags. These will appear in search engine results and help users understand what your site is about.
-
Internal Linking: Use internal links to help search engines crawl your website and improve the user experience by providing easy navigation.
-
Mobile Optimization: Google prioritizes mobile-friendly websites, so ensure your site works seamlessly on smartphones and tablets.
Build with Best Practices in Mind
By following these web development best practices, you’ll be able to create websites and applications that are fast, secure, user-friendly, and maintainable. Staying up to date with industry standards, prioritizing accessibility, and keeping security in mind will ensure that your work remains relevant and impactful for years to come.
Discuss Your Next Web Project with Us
Frontend & WordPress development made easy. We transform your ideas and designs into clean, functional code.